@dc42 yes, I logged that message right after the expansion boards got out of sync and the print shifted. I was also able to sniff the CAN messages of another print that failed in the same way and that's where I noticed 'lastTimeAcknowledgeDelay' being equal to zero for more than 10 times consecutively. That's how I started to test higher values for MaxTimeSyncDelay (initially 375), but I didn't know how far I could go.
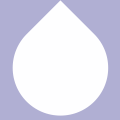
Posts made by leone
-
RE: [3.6.0-beta.2 and prev] Random out of sync
-
RE: [3.6.0-beta.2 and prev] Random out of sync
@dc42 I still have one:
All averaging filters OK Never used RAM 164992, free system stack 172 words Tasks: Move(3,nWait 7,0.0%,126) TMC(2,delaying,1.4%,51) HEAT(2,nWait 6,0.1%,89) CanAsync(5,nWait 4,0.0%,66) CanRecv(3,nWait 1,0.1%,73) CanClock(5,nWait 1,0.0%,63) LED(2,delaying,0.0%,123) ACCEL(3,nWait 6,0.0%,87) MAIN(1,running,97.4%,319) IDLE(0,ready,0.0%,29) AIN(2,delaying,1.0%,259), total 100.0% Owned mutexes: Last reset 02:15:02 ago, cause: power up Last software reset data not available Moves scheduled 37920, hiccups 0 (0.00/0.00ms), segs 37, step errors 0 (types 0x0), maxLate 0 maxPrep 62, ebfmin 0.00 max 0.00 Peak sync jitter -3/41, peak Rx sync delay 187, resyncs 1/0, no timer interrupt scheduled, next step interrupt due in 4247606105 ticks, disabled VIN voltage: min 24.2, current 24.4, max 24.5 MCU temperature: min 51.7C, current 58.0C, max 60.5C Driver 0: pos 12510580, 2056.0 steps/mm, standstill, SG min 0, read errors 0, write errors 0, ifcnt 25, reads 53750, writes 25, timeouts 0, DMA errors 0, CC errors 0 Last sensors broadcast 0x3fe0000000 found 9 225 ticks ago, 0 ordering errs, loop time 0 CAN messages queued 192060, send timeouts 0, received 305253, lost 0, ignored 16, errs 0, boc 0, free buffers 22, min 22, error reg 0 dup 0, oos 0/0/0/0, bm 0, wbm 0, rxMotionDelay 299, adv 35157/37138 Accelerometer: ADXL345, status: 00 I2C bus errors 0, naks 0, contentions 0, other errors 0
-
RE: [3.6.0-beta.2 and prev] Random out of sync
@dc42 with CanClockIntervalMillis = 200 the number of high acknowledge delays depends on how many clashes with other transmissions we have, and since it seems to happen quite random, the comparison with CanClockIntervalMillis = 199 is difficult to do. I tried to analyse 10 minutes in idle state with different configurations of CanClockIntervalMillis and MaxTimeSyncDelay.
I think I will run some prints with CanClockIntervalMillis = 199 and MaxTimeSyncDelay = 500, to see if the resync issue still occurs. From the analysys below it seems to be the one with less number of zeros and less high delays.
Do you think this is something relevant to include in the next release?freq199_thr300
Percentage of zeros: 0.50%
Percentage of values between 1 and 10: 96.60%
Percentage of values between 11 and 100: 1.13%
Percentage of values between 101 and 200: 1.10%
Percentage of values greater than 200: 0.67%freq200_thr500
Percentage of zeros: 0.00%
Percentage of values between 1 and 10: 93.77%
Percentage of values between 11 and 100: 2.30%
Percentage of values between 101 and 200: 2.10%
Percentage of values greater than 200: 1.83%freq199_thr500
Percentage of zeros: 0.00%
Percentage of values between 1 and 10: 96.63%
Percentage of values between 11 and 100: 1.30%
Percentage of values between 101 and 200: 1.10%
Percentage of values greater than 200: 0.97% -
RE: [3.6.0-beta.2 and prev] Random out of sync
@dc42 thanks for the suggestion!
From M122, "peak Tx sync delay" is about 480 both when the machine is idle and when is printing. This might correspond to the 600us needed to send the CANFD packet, right?
I tried with CanClockIntervalMillis = 199 and below is the (blue) plot from the CAN analyser. The interesting thing now is that the samples between ~4 and 300 are way more than before.
Then I also increased the MaxTimeSyncDelay to 500, and the number of zeros is considerably reduced, which I think is the goal to avoid resyncs.
Could this value of MaxTimeSyncDelay lead to other issues? -
[3.6.0-beta.1] Step timing error
Hi all,
I'm just reporting a step timing error I got with beta 1:2024-11-29 20:17:27 [warn] Error: Movement halted because a step timing error occurred (code 3). Please reset the controller. 2024-11-29 20:17:27 [warn] Error: Existing: start=159625402 length=7371, new: start=159630740, overlap=2033 time now=159629503
-
RE: [3.6.0-beta.2 and prev] Random out of sync
now I'm doing some tests increasing
StepTimer::MinSyncInterval
from 2000 to 4000. I noticed it was already increased some years ago, it might be the same issue, I am also running on a SAME70. -
RE: [3.6.0-beta.2 and prev] Random out of sync
Thanks @droftarts.
Update note: I got a resync also withMaxTimeSyncDelay = 375
. -
[3.6.0-beta.2 and prev] Random out of sync
Hi all,
from 3.6.0-beta.1 I started to have some layer shifts in the first layers of a print. These shifts happen randomly (the same gcode might shift in one print and not in the consecutive one, or just in another position). Still, I didn't find a way to replicate exactly the issue. The shift happens because the expansion boards go out of sync for about 2 seconds and the movement commands are discarded (as intended behavior from 3.6.0-beta.1).
So far, it never shifted when the bed compensation was disabled or, when enabled, it never shifted above the tapering height (5mm).
To debug, I connected a can sniffer device and analyzed the TimeSync messages. The out-of-sync happens when the fieldlastTimeAcknowledgeDelay
is zero for more than 10 consecutive times. From my understanding, the CanClockLoop doesn't update this variable if the previous message takes more thanMaxTimeSyncDelay
between the call of the send function and when the ISR actually processed the TX callback.From the plots you can see that the behavior of
lastTimeAcknowledgeDelay
with FW 3.5.2 and 3.6.0-beta.1 is similar, so I might never have had a shift before just because in 3.5.2 the movement commands weren't discarded.
I increasedMaxTimeSyncDelay
from 300 (400 usec?) to 375 (500 usec?) to see what happens: these spikes are still present but the number of zeros is reduced, so hopefully tolerant enough to never reach 10 consecutive samples.From the plot, it looks like the normal value for
lastTimeAcknowledgeDelay
is around 3-5 and a cloudy distribution around 250-300. So the original threshold of 300 would make sense.
I'm wondering why there are these spikes, after one spike to about 300 or more, the spike is then repeated every 5 samples but with a linear decreasing amplitude.
I tried also to disable the input shaping but the overall distribution is similar.Do you have any explanation for this behavior? Other processes or interrupts interfering? Do you think it would make sense to increase the
MaxTimeSyncDelay
threshold or is it just a patch? Do you foresee any issues arising from doing this?Thanks in advance.
-
RE: Version 3.6.0-beta.2 now available
Hi @chrishamm, I just noticed in the Git tree that the tag 3.6.0-beta.2 is pointing to a commit in the 3.5-dev branch (72d85117). Maybe the intended commit was 4cc23ddc in the 3.6-dev branch? Both have "Tidied up M307 processing" as message.
-
RE: [FW 3.5.2] M569.2 and microstepping lookup table
@dc42 great news!
The modulation parameter goes from -27 to 39. -
RE: [FW 3.5.2] M569.2 and microstepping lookup table
@dc42 unfortunately, the IDE doesn't say anything more than the datasheet/application notes.
My understanding here is that the sine wave is encoded as a list of increments/decrements to add to the START_SIN and START_SIN90 registries while increasing the microsteps. Since START_SIN and START_SIN90 represent the current of the two coils at the beginning of the electrical revolution, the value has to be greater or equal than zero.
It can be tuned for some desired behaviours or to make some weird configurations feasible (as in the picture below, where for offset less than 28 the wave is not feasible).
In general, from the examples in the datasheet and application notes, I saw the START_SIN registry always set to zero. -
RE: [FW 3.5.2] M569.2 and microstepping lookup table
@dc42 here they are. The sine offset should be "the absolute current at entry of the lookup table". It shifts up or down the sine wave, so to have it feasible I had to reduce the microstep amplitude. The maximum offset is not feasible, the higher it gets, the flatter the sinewave must be. Hope it's enough to clarify the behaviour, if you need something else, let me know.
-
RE: [FW 3.5.2] M569.2 and microstepping lookup table
@AndyE3D I followed the driver's datasheet and the application note 26:
https://www.analog.com/en/resources/app-notes/an-026.html
https://www.analog.com/media/en/technical-documentation/data-sheets/TMC5160A_datasheet_rev1.17.pdf
These are settings strongly dependent on the motor itself, and I have only nema24 motors. The configuration I posted is the best I found for this type of motor. -
RE: [FW 3.5.2] M569.2 and microstepping lookup table
@AndyE3D I am using the TMC5160 evaluation kit (https://www.mouser.de/ProductDetail/ADI-Trinamic/TMC5160-EVAL-KIT?qs=TiOZkKH1s2TJebvRqsmGsQ%3D%3D).
Once you connect the eval-kit to the pc you will find something called "sinus tool" and you can play with it. This is what in the user manual is called "microstepping look-up table"... I'm not sure if with "phase modulation" you're referring to the same thing. -
RE: [FW 3.5.2] High jerk good for circular path not for corners
@dc42 Thinking in abstract terms, to completely describe and have full control of such a transition, the total amount of parameters might be: max speed change, min speed change (square corner speed), angle threshold (to determine at which angle the curve has to drop), and the slope of the curve.
Focusing on the parameters and not on the type of curve now, I agree that for simplicity two parameters are preferable, but if we use max speed change and min speed change, the user doesn't have control over the transition between the two speeds. I think the slope of the curve can be hard coded (with a parameter or by the type of the curve itself), but if I had to choose a second parameter, between a min speed change or an angle threshold, I would rather prefer to have control on the angle threshold and have the min speed change decided by the FW (it might also be the minJerk). -
RE: [FW 3.5.2] High jerk good for circular path not for corners
@dc42 thanks for the feedback!
-
RE: [FW 3.5.2] High jerk good for circular path not for corners
@gloomyandy here it is. It's not much more complicate than the original DDA::MatchSpeed function. It can also be simplified by removing the rotation matrix definition and just compute the X coordinate of v2 in the reference frame of v1.
I also decided to implement it only for xy moves and keeping the possibility to disable it by setting the JerkDecay parameter to zero.void DDA::MatchSpeeds() noexcept { // v2 needs to be expressed in the reference frame of v1 // directionVector and next->directionVector are unit vectors // The rotation matrix R(-angle) can be expressed by using the directionVector[0] and directionVector[1] which are the X and Y components of v1 in the absolute reference frame (v1_0). const float rotationMatrix[2][2] = { { directionVector[0], directionVector[1] }, { -directionVector[1], directionVector[0] } }; // Project v2 in the reference frame of v1 (v2_1) const float nextVectorProjected[2] = { rotationMatrix[0][0] * next->directionVector[0] + rotationMatrix[0][1] * next->directionVector[1], rotationMatrix[1][0] * next->directionVector[0] + rotationMatrix[1][1] * next->directionVector[1] }; debugPrintf("JUNCTION: START targetNextSpeed [mm/s]: %f \n", (double)InverseConvertSpeedToMmPerSec(beforePrepare.targetNextSpeed)); DebugPrintVector("JUNCTION: directionVector \n", directionVector, 2); DebugPrintVector("JUNCTION: next->directionVector ", next->directionVector, 2); DebugPrintVector("JUNCTION: nextVectorProjected ", nextVectorProjected, 2); for (size_t drive = 0; drive < MaxAxesPlusExtruders; ++drive) { if (directionVector[drive] != 0.0 || next->directionVector[drive] != 0.0) { const float totalFraction = fabsf(directionVector[drive] - next->directionVector[drive]); const float jerk = totalFraction * beforePrepare.targetNextSpeed; float allowedJerk = reprap.GetPlatform().GetInstantDv(drive); if (flags.xyMoving && flags.isPrintingMove && reprap.GetPlatform().JerkDecay() != 0) { const float A = (reprap.GetPlatform().GetInstantDv(drive) - ConvertSpeedFromMmPerSec(MinimumJerk)); const float B = reprap.GetPlatform().JerkDecay(); const float C = ConvertSpeedFromMmPerSec(MinimumJerk); allowedJerk = A / (1 + (float)exp(B * (-nextVectorProjected[0] + reprap.GetPlatform().JunctionDeviation()))) + C; debugPrintf("JUNCTION: allowedJerk for drive %i: %f \n", drive, (double)InverseConvertSpeedToMmPerSec(allowedJerk)); } if (jerk > allowedJerk) { beforePrepare.targetNextSpeed = allowedJerk/totalFraction; } } } debugPrintf("JUNCTION: junctionSpeed [mm/s]: %f \n", (double)InverseConvertSpeedToMmPerSec(beforePrepare.targetNextSpeed)); }
-
RE: [FW 3.5.2] High jerk good for circular path not for corners
@gloomyandy your interpretation is correct. However, I have a couple of parameters that allow to change the behaviour of the curve.
The function is:allowedJerk = A / (1 + e^(B*(cosAngle + cosAngleThreshold))))+ C
where:
- A = maxJerk (defined by M566) - minJerk
- B = tunable parameter to define the slope of the decay from maxJerk to minJerk
- C = minJerk
Below the plot of the function with maxJerk = 600 mm/min, minJerk = 6 mm/min cosAngleThreshold = -0.707 (following the first picture, alpha=45deg) and increasing B.
In that configuration, vectors at 45deg will have an allowableJerk of 300mm/min, while for 90deg 6mm/min. I think having such a low jerk for sharp corners is not bad, also it allows the input shaping to work better since you have a bigger acceleration profile.
This approach gave me more flexibility, and with a proper setting of parameters you can have pretty much the same behaviour of the junction deviation. I can post the code of course if anyone is interested.
The weak point I see in both approaches (or working only inside DDA::MatchSpeeds) is when the round corners have a very fine mesh and the angle between two consecutive vectors is small. In that case, you may have the "illusion" of traveling a linear path while actually doing a circle and then end up going too fast.
-
RE: [FW 3.5.2] High jerk good for circular path not for corners
@oliof the possibility of having the s-curves sounds exciting! Really looking forward to it.
Meanwhile, I did some other tests to smooth the motion of the printer I'm currently working with.As @gloomyandy suggested, I tried to implement the grbl junction deviation algorithm inside the function DDA::MatchSpeed, but I wasn't able to get decent behavior out of it.
I believe the main reason why the junction deviation didn't perform well is because of the slow decreasing behavior in function of the angle, having again the problem of balancing the high jerk for arcs and the low jerks for corners.
So I improved my "adaptive jerk" approach, implementing a continuous function to scale down from the maximum to the minimum jerk value. I'm still under testing and tuning it but so far it seems to perform quite well. Below the difference of the junction deviation (tuned to have decent arcs) compared to the "adaptive jerk". (X axis is -cos(alpha) wrt the first sketch).